In the world of Android development, enabling seamless communication between apps is crucial. One of the most powerful tools for achieving this is the intent://
URL scheme. This system allows developers to create shortcuts and deep links that open specific features within an app or launch other apps directly.
This article provides an in-depth guide on how intent://
works, its key components, practical examples, and how to implement it securely.
What is intent://
in Android?
The intent://
scheme is a powerful feature in Android that developers can use to create deep links. Deep links allow users to access specific sections or features of an app directly, bypassing multiple layers of navigation. Additionally, intent://
URLs can launch other apps or execute actions like opening the settings menu, starting a phone call, or sending a text message.
This flexibility makes intent://
URLs a popular choice for:
- Creating custom app shortcuts.
- Integrating multiple apps for seamless workflows.
- Providing a better user experience by reducing unnecessary navigation.
How Does an intent://
URL Work?
An intent://
URL consists of multiple components that define what action to perform, what app or feature to launch, and how the system should handle the intent. Here’s a typical structure:
plaintextCopiar códigointent://host/#Intent;scheme=scheme_name;action=action_name;category=category_name;component=component_name;end;
Let’s break down these components:
intent://host/
: Specifies the scheme and the optional host. This part tells the system that it’s dealing with an intent.scheme=scheme_name
: Defines the URL scheme, such ashttp
,mailto
, or a custom app-specific scheme.action=action_name
: Indicates the action to perform, such asandroid.intent.action.VIEW
for opening a webpage.category=category_name
: Adds a category to the intent, such asandroid.intent.category.DEFAULT
.component=component_name
: Optionally specifies the app or component to handle the intent.end;
: Marks the end of the intent.
This modular structure allows developers to customize intents for various use cases.
Practical Examples of intent://
in Android
Below are some practical examples of how to use intent://
URLs to perform common tasks:
1. Open the Phone’s Settings
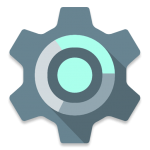
To open the settings menu on an Android device, you can use the following intent URL:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://settings/#Intent;scheme=android-app;action=android.settings.SETTINGS;end;
Use Case: This can be useful in apps that require users to change permissions or enable certain features.
2. Open the Phone’s Accessibility
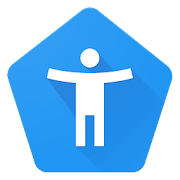
To open the settings menu on an Android device, you can use the following intent URL:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://com.google.android.accessibility.switchaccess/#Intent;scheme=android-app;end
Use Case: Apps focused on accessibility can guide users to these settings for configuration.
3. Launch Google Chrome
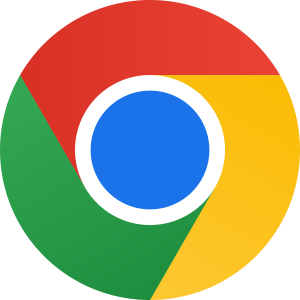
To open Google Chrome with a specific website:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://www.google.com/#Intent;scheme=https;package=com.android.chrome;end;
Use Case: This is useful for apps that need to open specific webpages while ensuring the Chrome browser handles the request.
4. Open the Play Store
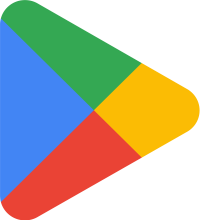
To open a specific app page in the Google Play Store:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://details?id=com.whatsapp#Intent;scheme=market;action=android.intent.action.VIEW;end;
Use Case: This is great for apps that recommend other apps or guide users to download companion software.
5.Launch Moto Secure
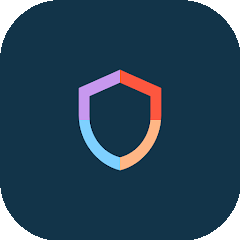
To open a specific app page in the Google Play Store:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://com.motorola.securityhub/#Intent;scheme=android-app;end
6.Launch Moto
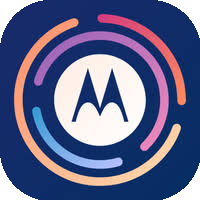
To open a specific app page in the Google Play Store:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://com.motorola.moto/#Intent;scheme=android-app;end
7.Launch Security Xiaomi (HyperOS & Miui)
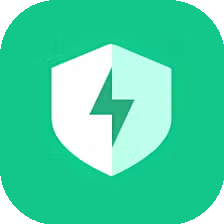
To open a specific app page in the Google Play Store:
Click the buttons below to test how intent:// works. These are educational examples and may not work on all devices.
plaintextCopiar códigointent://com.miui.securitycenter/#Intent;scheme=android-app;end
Use Case: Useful for apps that involve device optimization or security.
Benefits of Using intent://
- Enhanced User Experience: By reducing navigation time, users can access features or settings faster.
- Seamless Integration: Apps can interact with each other effortlessly.
- Customization: Developers can define highly specific actions using intent components.
Best Practices for Using intent://
1. Validate Input
Always validate data received through intents to prevent malicious activity. For example:
- Check if the data matches the expected format.
- Reject unknown or potentially harmful data.
2. Restrict Access
Use <intent-filter>
in your AndroidManifest.xml
to control which apps can handle your intents. Example:
xmlCopiar código<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="https" android:host="www.example.com" />
</intent-filter>
</activity>
3. Avoid Overprivileged Intents
Do not create intents that perform overly sensitive actions without proper user consent.
4. Add Contextual Guidance
Always inform users about the purpose of the intent and what it does. For instance:
«This button will open your device settings. Please adjust permissions as needed.»
Security Considerations
When implementing intent://
, prioritize user safety:
- Explain Intent Actions: Clearly state what each example does and its purpose.
- Test Across Devices: Ensure intents behave consistently on different Android versions and device manufacturers.
Conclusion
intent://
URLs are a powerful tool for creating deep links, shortcuts, and app integrations in Android development. Whether you’re opening the settings menu, launching Chrome, or guiding users to the Play Store, these URLs simplify user interactions and improve app usability.
However, with great power comes great responsibility. Always validate inputs, restrict access, and prioritize user safety. By following best practices, you can leverage intent://
URLs to their full potential while maintaining a secure and user-friendly environment.
If you’re an Android developer, now is the time to experiment with intent://
and elevate the functionality of your apps. Happy coding!